Environment Variables in React
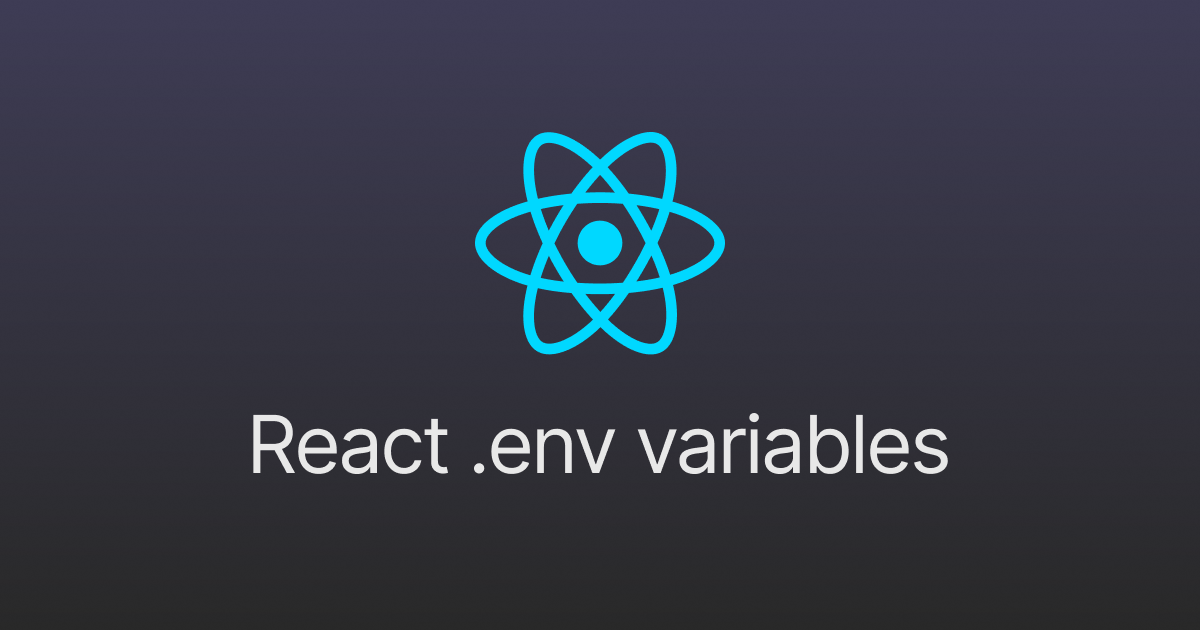
Setting Up & Using Environment Variables in React
-
Create an Environment File
Create a
.env
file in the root of your project. -
Define Your Variables
Inside the
.env
file, define your variables. React requires variable names to start withREACT_APP_
. For example:Copy1REACT_APP_BASE_URL=https://www.example.com
-
Accessing Variables in Code
In your React components or JavaScript files, access these variables using
process.env.
For example:Copy1const baseUrl = process.env.REACT_APP_BASE_URL;
Note: React also supports other built-in environment files like
.env.local
,.env.development
,.env.production
, and their.local
variants. For more complex setups, consider usingenv-cmd
for easier management. Refer to the React environment variables guide for more details.
Using env-cmd for Environment Management
env-cmd
simplifies managing multiple environment files by allowing you to specify which environment file to use when running your scripts.
Steps to Set Up and Use
-
Install
env-cmd
First, add
env-cmd
to your project.Copy1npm install env-cmd --save-dev
-
Create Environment Files
Create
.env.dev
and.env.prod
files in the root of your project with the appropriate content..env.dev
Copy1REACT_APP_BASE_URL=https://www.dev.example.com
.env.prod
Copy1REACT_APP_BASE_URL=https://www.prod.example.com
-
Update Scripts in
package.json
Modify the
scripts
section in yourpackage.json
to useenv-cmd
for different environments.Copy1"scripts": { 2 "start": "env-cmd -f .env.dev react-scripts start", 3 "build:dev": "env-cmd -f .env.dev react-scripts build", 4 "build:prod": "env-cmd -f .env.prod react-scripts build", 5 "test": "env-cmd -f .env.dev react-scripts test" 6}
This setup ensures that
env-cmd
loads the appropriate environment file depending on the script you run. -
Run Your Scripts
Use the following commands to run your project with the specified environment:
- Development (Start)
- Development (Build)
- Production (Build)
- Testing
env-cmd
will automatically use the variables defined in.env.dev
or.env.prod
based on the script being executed.
Explanation
When running the development start command, env-cmd
will use the .env.dev
file and make the environment variables available to your React application. Similarly, running the development build command will use .env.dev
, and the production build command will use .env.prod
.
Best Practices
- Do Not Commit
.env
Files: Add.env
to your.gitignore
file to prevent sensitive information from being committed to your repository. - Use Environment Variables for Configuration Only: Avoid storing secrets or sensitive information directly in your
.env
files. Use secure vault services for sensitive data to reduce the risk of exposure. - Consistent Naming: Keep your variable names consistent and descriptive to avoid confusion.
Security Considerations
- Avoid Storing API Keys Directly: Storing API keys and other secrets directly in your
.env
files can be risky because these files might be exposed if your source control is compromised or if the files are inadvertently shared. Instead, use environment variables to reference keys stored in secure vault services. - Minimize Exposure in Client-Side Code: Be cautious when using environment variables in client-side code. Any variable included in your React app's code can be accessed by users. Only include non-sensitive configuration settings in your React environment variables.
By following these steps, you can efficiently manage environment-specific settings in your React applications. This setup helps maintain clean code and secure configurations.