React Context API
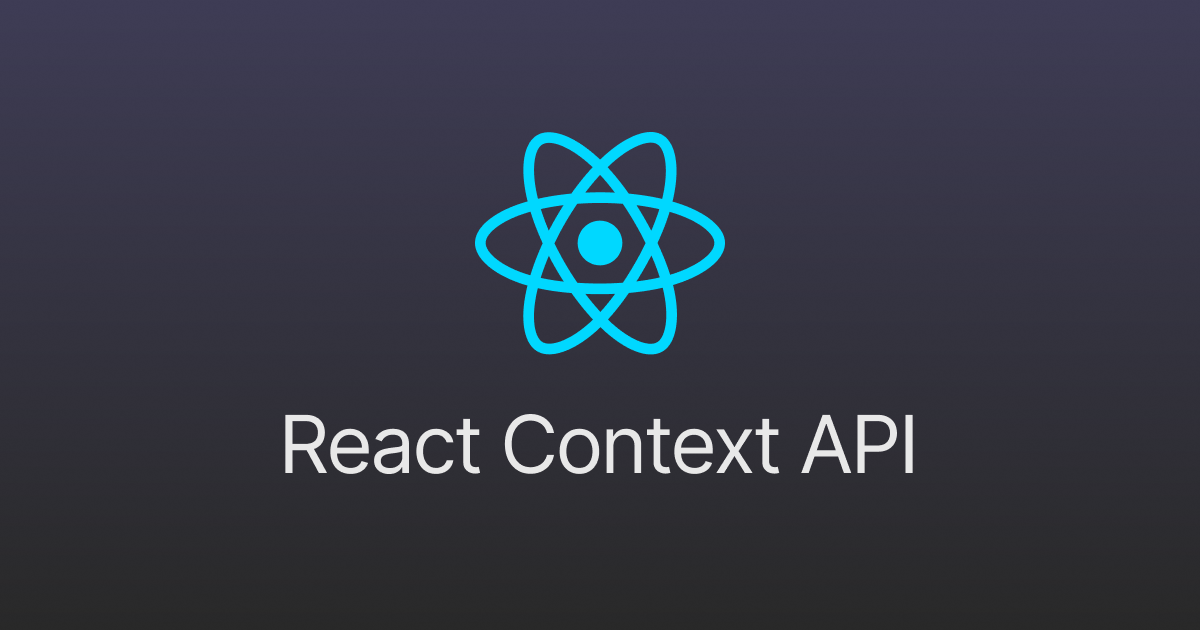
Understanding the React Context API: When and How to Use It
The React Context API is a powerful tool for managing state in React applications. While it provides a straightforward way to pass data through the component tree without prop drilling, understanding when and how to use it effectively is key. In this article, we’ll explore what the Context API is, how it differs from libraries like Redux, and when it’s the right (or wrong) tool for the job.
What Is the React Context API?
The React Context API allows you to create global data that can be accessed anywhere in your component tree without having to pass props down manually at each level. It is part of the core React library, making it easy to use without any additional installations.
Context is often used for sharing data that doesn’t change frequently, such as theme, language preferences, or authentication status. It works through two main components:
- Provider: Supplies the data to all child components.
- Consumer: Allows components to access the provided data.
When to Use the Context API
The Context API is ideal for scenarios where you need to:
- Share state across many nested components.
- Avoid prop drilling (i.e., passing props down through multiple layers of components).
- Provide a global state for static or rarely-changing data like theme, language, or user authentication.
Example: Managing User Preferences
In this example, we'll manage user preferences such as language and notification settings using the React Context API. We’ll create a context for storing the user preferences, allow updating these preferences, and use them across the app.
Step 1: Create the PreferencesContext.tsx
File
This file contains the context provider and custom hook for managing preferences.
Copy1// PreferencesContext.tsx 2import React, { createContext, useContext, useState, ReactNode } from 'react'; 3 4interface PreferencesData { 5 language: string; 6 notificationsEnabled: boolean; 7} 8 9interface PreferencesContextType { 10 preferences: PreferencesData; 11 updatePreferences: (preferences: PreferencesData) => void; 12} 13 14const PreferencesContext = createContext<PreferencesContextType | undefined>(undefined); 15 16export const PreferencesProvider = ({ children }: { children: ReactNode }) => { 17 const [preferences, setPreferences] = useState<PreferencesData>({ 18 language: 'en', 19 notificationsEnabled: true, 20 }); 21 22 const updatePreferences = (preferences: PreferencesData) => { 23 setPreferences(preferences); 24 }; 25 26 return ( 27 <PreferencesContext.Provider value={{ preferences, updatePreferences }}> 28 {children} 29 </PreferencesContext.Provider> 30 ); 31}; 32 33export const usePreferences = () => { 34 const context = useContext(PreferencesContext); 35 if (!context) { 36 throw new Error('usePreferences must be used within a PreferencesProvider'); 37 } 38 return context; 39};
Step 2: Create the PreferencesDisplay.tsx
File
This file is for displaying the current user preferences.
Copy1// PreferencesDisplay.tsx 2import React from 'react'; 3import { usePreferences } from './PreferencesContext'; 4 5const PreferencesDisplay = () => { 6 const { preferences } = usePreferences(); 7 8 return ( 9 <div> 10 <h2>User Preferences</h2> 11 <p>Language: {preferences.language}</p> 12 <p>Notifications: {preferences.notificationsEnabled ? 'Enabled' : 'Disabled'}</p> 13 </div> 14 ); 15}; 16 17export default PreferencesDisplay;
Step 3: Create the PreferencesForm.tsx
File
This file is for handling the form to update user preferences.
Copy1// PreferencesForm.tsx 2import React, { useState } from 'react'; 3import { usePreferences } from './PreferencesContext'; 4 5const PreferencesForm = () => { 6 const { updatePreferences } = usePreferences(); 7 const [language, setLanguage] = useState(''); 8 const [notificationsEnabled, setNotificationsEnabled] = useState(false); 9 10 const handleSubmit = (e: React.FormEvent) => { 11 e.preventDefault(); 12 updatePreferences({ language, notificationsEnabled }); 13 }; 14 15 return ( 16 <form onSubmit={handleSubmit}> 17 <h2>Update Preferences</h2> 18 <div> 19 <label> 20 Language: 21 <input 22 type="text" 23 placeholder="Enter language" 24 value={language} 25 onChange={(e) => setLanguage(e.target.value)} 26 /> 27 </label> 28 </div> 29 <div> 30 <label> 31 Enable Notifications: 32 <input 33 type="checkbox" 34 checked={notificationsEnabled} 35 onChange={() => setNotificationsEnabled(!notificationsEnabled)} 36 /> 37 </label> 38 </div> 39 <button type="submit">Update Preferences</button> 40 </form> 41 ); 42}; 43 44export default PreferencesForm;
Step 4: Modify the App.tsx
File
The App.tsx file brings all the components together.
Copy1// App.tsx 2import React from 'react'; 3import { PreferencesProvider } from './PreferencesContext'; 4import PreferencesDisplay from './PreferencesDisplay'; 5import PreferencesForm from './PreferencesForm'; 6 7const App = () => { 8 return ( 9 <PreferencesProvider> 10 <div style={{ padding: '20px' }}> 11 <h1>React Context API - Preferences Example</h1> 12 <PreferencesDisplay /> 13 <PreferencesForm /> 14 </div> 15 </PreferencesProvider> 16 ); 17}; 18 19export default App;
When Not to Use the Context API
Although the Context API is versatile, it’s not suitable for every global state management scenario. It can have limitations, such as:
- Frequent State Changes: Context is not optimized for managing rapidly changing or complex state. Re-renders are triggered every time the provider’s value changes, potentially leading to performance issues.
- Complex State Management: If your application state is highly structured and involves actions or effects (e.g., API calls, caching, or optimistic updates), a more advanced library like Redux or TanStack Query may be more appropriate.
How Context Differs from Redux and Other State Management Libraries
While Context provides a simple way to share state, Redux and other state management libraries (like Zustand or MobX) offer more robust solutions:
- State Management Complexity: Redux excels at handling complex, nested, and normalized state structures, along with sophisticated actions and reducers.
- Middleware Support: Redux can integrate middleware (e.g., Redux Thunk or Redux Saga) for handling asynchronous operations.
- DevTools and Debugging: Redux offers powerful DevTools for time-travel debugging and visualizing state changes.
- Selective Subscriptions: Redux allows components to subscribe to specific parts of the state, reducing unnecessary re-renders.
By comparison, the Context API:
- Is simpler and built into React, with no need for external dependencies.
- Doesn’t have built-in mechanisms for actions, reducers, or middleware.
- Triggers re-renders for all consumers whenever the context value changes.
When to Use Redux Over Context
- Complex State Logic: If your application state involves multiple actions, reducers, or interactions.
- Performance Considerations: For applications with frequent state updates and performance-critical components.
- Asynchronous Workflows: Redux handles async operations more effectively with its middleware support.
When Context Is the Right Choice
- Simpler State Sharing: Context is ideal for small to medium-sized applications or for managing static/global state.
- Avoiding Overhead: Context is easier to implement without the learning curve of Redux or the overhead of installing additional libraries.
Conclusion
The React Context API is a flexible tool for managing global state in your application. It’s particularly well-suited for static or infrequently changing data like themes or authentication status. However, for more complex or performance-critical applications, libraries like Redux offer more robust solutions.
By understanding the strengths and limitations of Context, you can make informed decisions about when to use it and when to opt for more advanced state management libraries.