React Controlled vs Uncontrolled
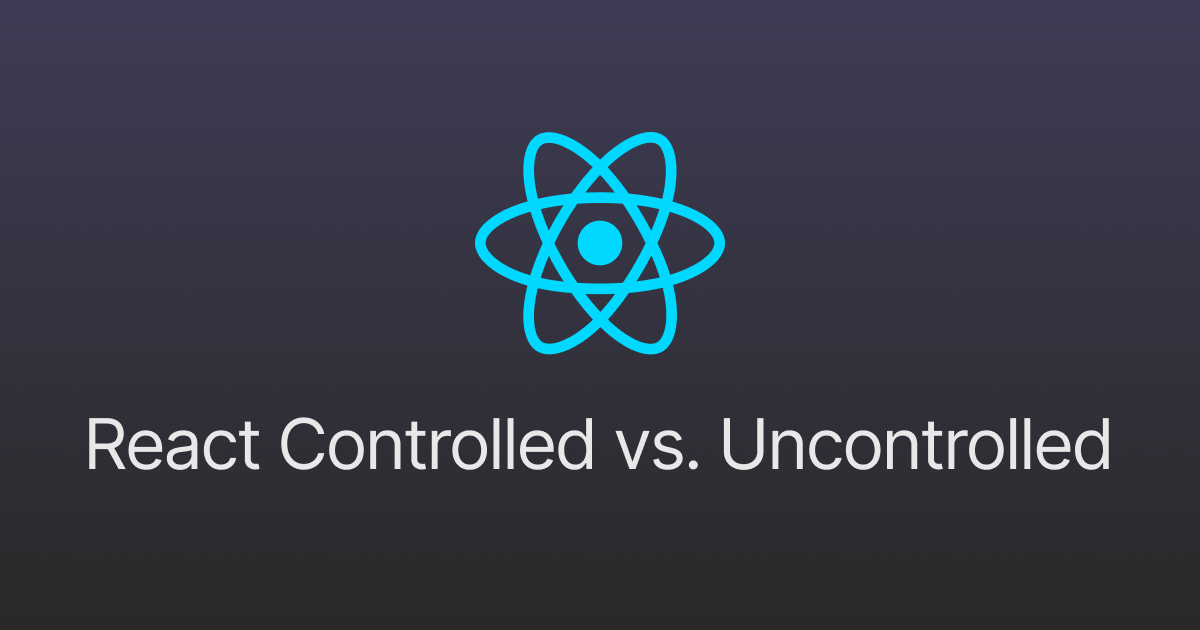
React Controlled vs. Uncontrolled Components
When working with forms in React, understanding the difference between controlled and uncontrolled components is essential. Both approaches have their use cases and can affect your application's performance, behavior, and development experience. This article will break down these two concepts and help you decide when to use each.
Controlled Components
A controlled component in React is a form element that is fully managed by the component's state. Changes to the input are handled by updating the state, and the value is passed to the input element through props.
Example of a Controlled Component (TSX)
Copy1import React, { useState } from 'react'; 2 3const ControlledInput: React.FC = () => { 4 const [inputValue, setInputValue] = useState<string>(''); 5 6 const handleChange = (event: React.ChangeEvent<HTMLInputElement>) => { 7 setInputValue(event.target.value); 8 }; 9 10 const handleSubmit = (event: React.FormEvent<HTMLFormElement>) => { 11 event.preventDefault(); 12 alert(`Submitted value: ${inputValue}`); 13 }; 14 15 return ( 16 <form onSubmit={handleSubmit}> 17 <label htmlFor="name">Name:</label> 18 <input 19 type="text" 20 id="name" 21 value={inputValue} 22 onChange={handleChange} 23 /> 24 <button type="submit">Submit</button> 25 </form> 26 ); 27}; 28 29export default ControlledInput;
Characteristics of Controlled Components
- State Management: The component manages the form state explicitly.
- Two-Way Data Binding: Any change to the input is reflected in the state, and the state drives the input's value.
- Validation: Real-time validation is easier to implement because you have control over each keystroke.
Real-World Example
A search bar that filters a list of items as the user types would typically use a controlled component to provide real-time feedback.
Advantages
- Easier to implement complex form validation.
- Ensures data consistency between the UI and the state.
- Provides more control over user input behavior.
Disadvantages
- Can become verbose and complex for forms with many fields.
Uncontrolled Components
Uncontrolled components rely on the DOM to manage form values. Instead of binding the input value to state, you retrieve the value when needed using a ref
.
Example of an Uncontrolled Component (TSX)
Copy1import React, { useRef } from 'react'; 2 3const UncontrolledInput: React.FC = () => { 4 const inputRef = useRef<HTMLInputElement>(null); 5 6 const handleSubmit = (event: React.FormEvent<HTMLFormElement>) => { 7 event.preventDefault(); 8 if (inputRef.current) { 9 alert(`Submitted value: ${inputRef.current.value}`); 10 } 11 }; 12 13 return ( 14 <form onSubmit={handleSubmit}> 15 <label htmlFor="name">Name:</label> 16 <input type="text" id="name" ref={inputRef} /> 17 <button type="submit">Submit</button> 18 </form> 19 ); 20}; 21 22export default UncontrolledInput;
Characteristics of Uncontrolled Components
- No State Management: The form element manages its own state.
- Access via Refs: You use
refs
to read the input's value when needed. - Simpler Implementation: No need to handle
onChange
events to update state.
Real-World Example
A file upload form where the value of the input is only needed at the time of submission might use an uncontrolled component.
Advantages
- Simpler to set up for basic forms.
- Can be more performant for forms that don’t require real-time validation.
Disadvantages
- Harder to implement validation and error handling.
- Limited control over the input's state.
Key Differences
Feature | Controlled Components | Uncontrolled Components |
---|---|---|
State Management | Managed in React component state | Managed by the DOM |
Value Binding | Value prop is set by state | Value retrieved via ref |
Validation | Easier to implement | Harder to implement |
Complexity | More verbose | Simpler for basic use cases |
Performance | Slightly slower due to state updates | Can be faster for simple forms |
When to Use Each
-
Use Controlled Components when:
- You need real-time validation or user feedback.
- You want strict control over the form state.
- The form is complex and has many dependent fields.
- Example: A signup form with password strength indicators and live feedback for valid email addresses.
-
Use Uncontrolled Components when:
- You need quick, simple forms without much validation.
- You want better performance and don't need to handle input changes frequently.
- The form is used infrequently or as a one-off.
- Example: A search form that only needs the value on submission.
Conclusion
Both controlled and uncontrolled components have their pros and cons. Controlled components offer better control and are well-suited for complex forms with real-time feedback, while uncontrolled components are simpler and faster for basic forms. By understanding their differences, you can choose the best approach for your React application's specific needs.